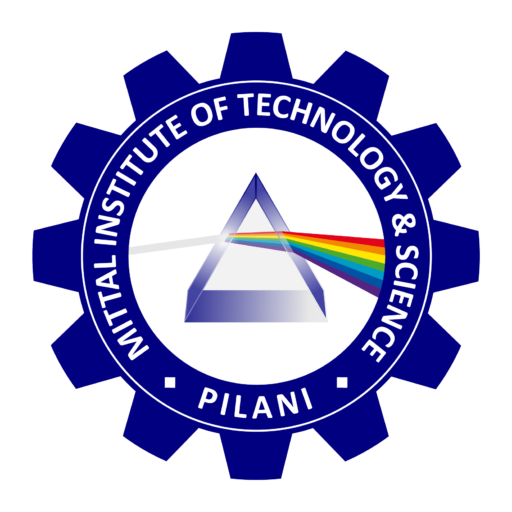
Components and Steps in the Development of a Web Application Using Java
The development of a web application involves multiple components and follows a well-defined process, particularly when Java is used as the primary technology. Java has been a popular choice for building robust, scalable, and secure web applications due to its platform independence, extensive libraries, and frameworks. This essay outlines the main components and steps involved in developing a web application using Java.
Components of a Java Web Application
- Client-Side Components
- HTML/CSS/JavaScript: The front-end of the web application consists of HTML (Hypertext Markup Language) for structuring web pages, CSS (Cascading Style Sheets) for styling, and JavaScript for adding dynamic behavior. Java-based web frameworks, such as JSP (JavaServer Pages) or Thymeleaf, can generate dynamic HTML content.
- Browser: The browser acts as the client interface that interacts with the server by sending requests (through URLs) and rendering responses (HTML, CSS, JavaScript).
- Server-Side Components
- Servlets: Java servlets are the backbone of Java web applications. They are Java programs that run on the server, handle client requests, process business logic, and send responses. Servlets follow a request-response model and communicate with the client using the HTTP protocol.
- JavaServer Pages (JSP): JSP is a server-side technology that allows the embedding of Java code within HTML to generate dynamic web content. JSP files are compiled into servlets by the server and provide an efficient way to create views in MVC (Model-View-Controller) architecture.
- JavaBeans/POJOs: These are Plain Old Java Objects that represent business logic and data in the application. They are used to encapsulate data and provide methods for manipulating it.
- Application Server: Java web applications are typically deployed on an application server (such as Apache Tomcat, JBoss, or GlassFish). The server manages the life cycle of servlets, handles requests, manages sessions, and provides various other services like connection pooling, security, etc.
- Database Components
- Database Management System (DBMS): Web applications often require persistent storage of data, which is handled by a DBMS (such as MySQL, PostgreSQL, or Oracle). Java web applications use JDBC (Java Database Connectivity) or ORM (Object-Relational Mapping) frameworks like Hibernate to communicate with the database.
- Database Connectivity Layer (JDBC/ORM): This layer manages data retrieval, insertion, updating, and deletion between the web application and the database.
- Middleware Components
- Frameworks: Java frameworks like Spring and Hibernate simplify development by providing built-in functionalities, security, and integration features. Spring MVC is widely used for managing the MVC architecture in web applications.
- Security: Java provides various security features like authentication, authorization, and encryption through frameworks like Spring Security or Java EE’s built-in security mechanisms. It helps ensure the safety of sensitive data and the overall application.
- Web Services: Java applications often expose or consume web services, which can be built using RESTful (JAX-RS) or SOAP-based (JAX-WS) APIs.
Steps in Developing a Web Application Using Java
- Requirement Gathering and Planning
- The development process starts with understanding the business requirements and functional specifications. This stage involves discussions with stakeholders, defining the scope, and preparing the technical roadmap.
- Planning includes selecting the appropriate technology stack, deciding on the application architecture (e.g., layered architecture or microservices), and preparing the development and deployment environments.
- Designing the Architecture
- The next step is to design the architecture of the web application. Java-based applications often follow the MVC (Model-View-Controller) design pattern:
- Model: Represents the application’s data and business logic.
- View: The UI components that the user interacts with.
- Controller: Handles user inputs and coordinates between the model and view.
- System components, such as data flow, user interactions, external integrations, and security measures, are also planned.
- The next step is to design the architecture of the web application. Java-based applications often follow the MVC (Model-View-Controller) design pattern:
- Setting up the Development Environment
- Install and configure necessary tools and environments for development. Key components include:
- IDE: Integrated Development Environment like Eclipse or IntelliJ IDEA for writing, compiling, and debugging Java code.
- Build Tools: Tools like Maven or Gradle for managing dependencies and automating the build process.
- Version Control: Git is widely used for version control to track code changes and manage collaboration.
- Install and configure necessary tools and environments for development. Key components include:
- Writing the Code
- Front-End Development: Design the user interface (UI) using HTML, CSS, and JavaScript. Use JSP or Thymeleaf to integrate dynamic Java code into the views.
- Back-End Development: Write servlets, controllers, and service classes to implement business logic. Implement the database access layer using JDBC or an ORM framework like Hibernate.
- Form Validation: Implement client-side and server-side form validation using JavaScript and Java-based frameworks.
- Database Design
- Design the database schema based on the data requirements of the application. Create tables, define relationships, and write SQL queries or use Java Persistence API (JPA) to interact with the database.
- Set up JDBC or ORM to manage database transactions and ensure efficient data operations.
- Implementing Security
- Implement security measures to protect the application from common vulnerabilities like SQL injection, cross-site scripting (XSS), and cross-site request forgery (CSRF).
- Use encryption for sensitive data, ensure secure authentication and authorization mechanisms, and apply SSL/TLS for secure communication.
- Testing
- Perform unit testing for individual components using JUnit or other testing frameworks.
- Conduct integration testing to ensure all components work together seamlessly.
- Carry out user acceptance testing (UAT) to verify the application meets the business requirements.
- Load testing and stress testing are also important to evaluate the performance and scalability of the application.
- Deployment
- Once the application passes testing, it is packaged into a deployable format (e.g., WAR or JAR file) and deployed on an application server (like Tomcat or JBoss) in a production environment.
- The deployment process includes configuring the server, setting up databases, and ensuring the application is accessible to users.
- Maintenance and Updates
- After deployment, ongoing maintenance is required to fix bugs, address security vulnerabilities, and make performance improvements.
- Periodically update the application to meet evolving business needs, add new features, and support new technologies.
The development of a web application using Java involves a combination of client-side and server-side technologies, frameworks, and databases. By following a systematic approach—from gathering requirements to deployment and maintenance—Java developers can create powerful and scalable web applications. Java’s ecosystem of tools, libraries, and frameworks provides the flexibility and capability to build applications that meet modern web standards.