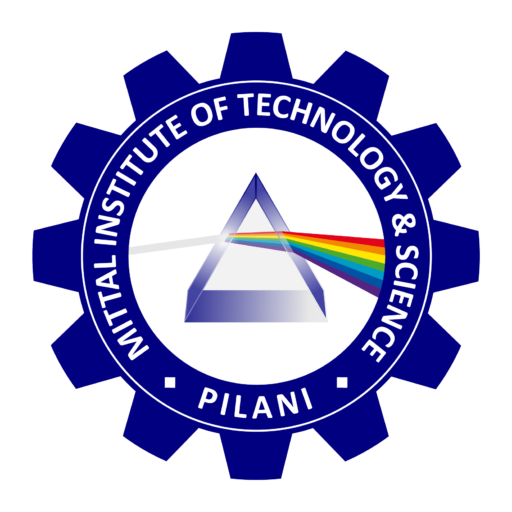
Compiler Construction
Compiler construction is a key field in computer science that bridges the gap between high-level programming languages and the machine-level code that computers execute. A compiler is a software tool that translates code written in a high-level language (like C, Java, or Python) into a lower-level language, typically machine code or assembly language, enabling the computer to understand and execute the program. The process of compiler construction involves several phases and requires an understanding of both programming languages and computer architecture.
Phases of Compiler Construction
The process of compiling a program is generally divided into two broad categories: analysis and synthesis. These categories are further divided into a series of distinct phases, which work together to produce an executable program from source code.
1. Lexical Analysis
The first phase of compilation is lexical analysis, also called scanning. This phase involves breaking the input program into a sequence of tokens, which are atomic units of the program’s source code, such as keywords, identifiers, operators, and symbols. Lexical analysis uses regular expressions to define patterns for different tokens, which are then recognized by a finite automaton.
The output of this phase is a stream of tokens that the next phase can process. Lexical analysis also removes white spaces, comments, and groups together meaningful sequences of characters.
2. Syntax Analysis
The second phase is syntax analysis, also called parsing. In this phase, the stream of tokens generated by the lexical analyzer is examined to check if they form a syntactically correct sequence according to the grammar of the programming language. A parse tree (or syntax tree) is constructed, which represents the hierarchical structure of the source program.
This phase ensures that the source program follows the correct structure defined by the language’s grammar. Context-free grammars (CFG) are often used to describe the syntax of programming languages. Syntax analysis can be achieved using different parsing techniques such as top-down (e.g., LL parsers) or bottom-up (e.g., LR parsers).
3. Semantic Analysis
In semantic analysis, the parse tree from the syntax analysis phase is checked for semantic consistency with the language’s rules. This phase verifies aspects like type checking, scope resolution, and the correct use of variables and functions. The compiler ensures that operations are performed on compatible data types and that variable declarations match their use within the code.
For instance, trying to add a string to an integer might pass through syntax analysis but will be flagged as an error during semantic analysis. The result of this phase is often an annotated syntax tree with additional information for later phases.
4. Intermediate Code Generation
The intermediate code generation phase translates the source code into an intermediate representation (IR). This code is neither machine-specific nor too high-level, acting as a bridge between the source code and the final machine code. Examples of IRs include three-address code, abstract syntax trees (AST), and static single assignment (SSA) form.
Intermediate code provides an abstraction that allows for machine-independent optimization and easier code generation. It’s a crucial step because it decouples the front-end of the compiler (which processes the high-level language) from the back-end (which generates machine code for a specific architecture).
5. Optimization
In the optimization phase, the intermediate code is transformed into a more efficient version. The goal of optimization is to improve the performance of the code, reduce its size, or both, without altering its functionality. Optimizations can be machine-independent, such as dead code elimination, loop unrolling, and constant folding, or machine-specific, such as instruction selection and register allocation.
Optimization can be performed at different levels—source code optimization, intermediate code optimization, or target code optimization. However, excessive optimization can make the code harder to debug and may even introduce subtle bugs.
6. Code Generation
The code generation phase converts the optimized intermediate representation into the target machine code. This involves mapping high-level constructs (such as loops and conditionals) into low-level instructions (like jumps and comparisons) that a computer’s CPU can execute. The code generator takes into account the architecture of the target machine, including its instruction set, registers, and memory model.
The generated machine code must be efficient in terms of both space and time, as well as compatible with the hardware. During this phase, the compiler may also perform tasks such as instruction scheduling and register allocation to ensure that the program runs efficiently on the target machine.
7. Code Linking
Once machine code is generated, it may still depend on other modules, libraries, or system calls. The code linking phase handles this by linking together all the object files and libraries into a single executable program. This step ensures that all references to external functions or variables are resolved.
Components of a Compiler
A compiler typically consists of several components, each responsible for a specific task in the compilation process. These include:
- Scanner: Performs lexical analysis.
- Parser: Carries out syntax analysis.
- Semantic Analyzer: Handles semantic checks.
- Intermediate Code Generator: Translates high-level code into an intermediate form.
- Optimizer: Improves the intermediate code’s performance.
- Code Generator: Produces target machine code.
- Error Handler: Detects and reports errors in each phase.
Types of Compilers
- Single-Pass Compiler: A compiler that completes all phases in one pass over the source code. It is typically faster but less powerful in terms of optimizations.
- Multi-Pass Compiler: A compiler that performs multiple passes over the source code, allowing for more sophisticated optimizations.
- Just-In-Time (JIT) Compiler: A compiler that translates code at runtime, commonly used in virtual machines like the JVM for Java.
- Cross Compiler: A compiler that generates code for a platform different from the one it runs on.
Conclusion
Compiler construction is a complex but fundamental aspect of computer science, playing a vital role in the software development process. It involves breaking down a high-level program into tokens, verifying its syntax and semantics, generating efficient intermediate code, optimizing it, and finally producing machine code. A well-designed compiler can significantly improve the performance and portability of a program, making compiler construction a critical area of research and innovation in computing.
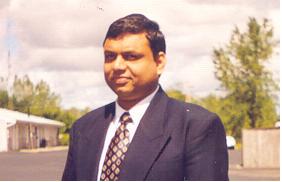
Professor Rakesh Mittal
Computer Science
Director
Mittal Institute of Technology & Science, Pilani, India and Clearwater, Florida, USA